Implementing Reorder In Ionic 4

Software Developer | Technical Writer | Actively helping users with their questions on Stack Overflow.
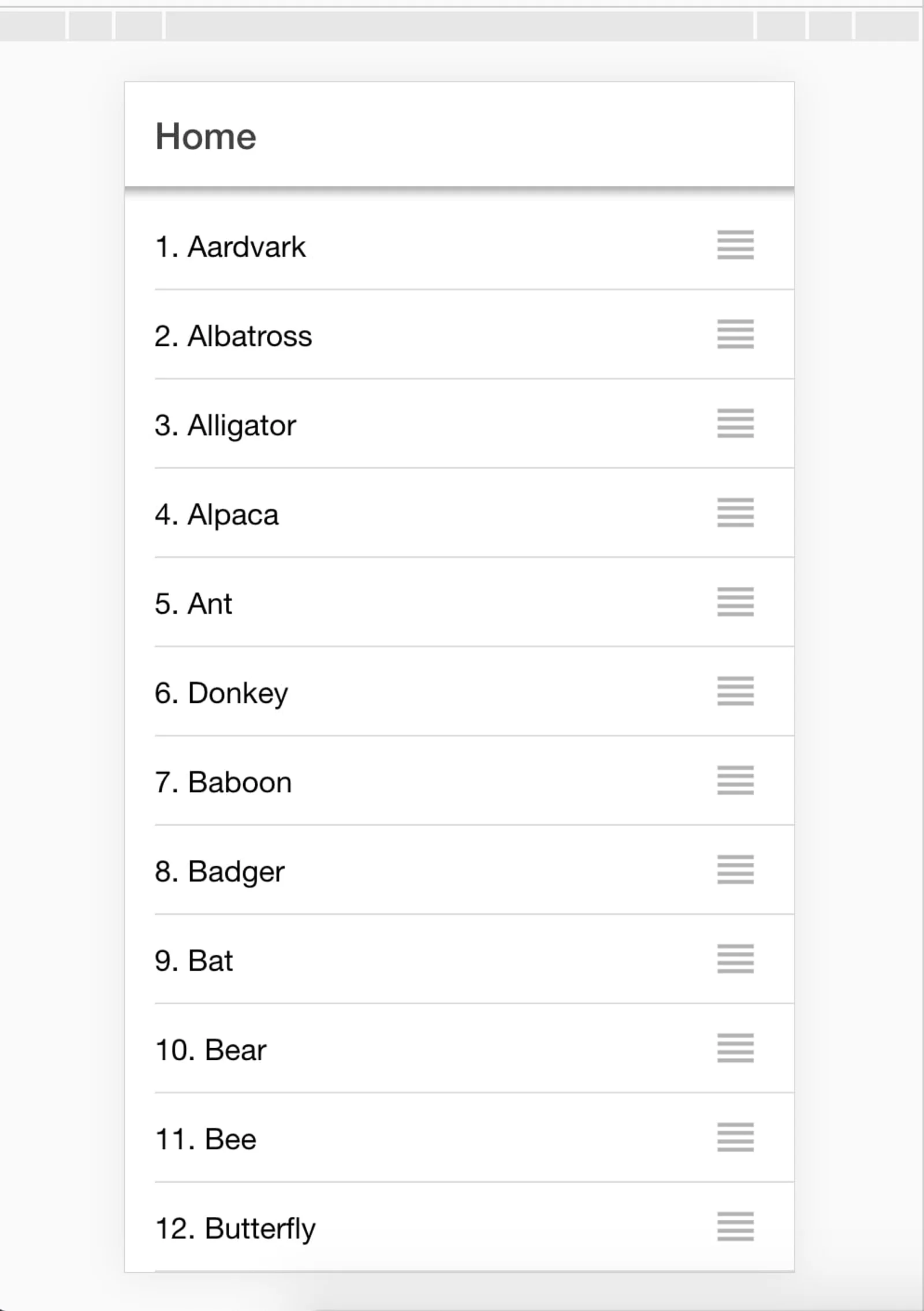
The ion-reorder component will allow you to drag and drop items, thus changing the order of the list.
Reordering Items Using Ion-Reorder
Ionic provides a reorder component called <ion-reorder>
, that will allow users to changes the order of the list. The <ion-reorder>
needs to be used inside the <ion-reorder-group>
, which will act as a wrapper for the items inside <ion-reorder>
.
First, we need to create an array to add items in the list, in this tutorial I used an array containing different animal names.
Example:
this.animals =
[
"1. Aardvark",
"2. Albatross",
"3. Alligator",
"4. Alpaca",
"5. Ant",
"6. Donkey",
"7. Baboon",
"8. Badger",
"9. Bat",
"10. Bear",
"11. Bee",
"12. Butterfly",
"13. Camel",
"14. Chicken",
"15. Cockroach",
"16. Horse",
]
Then in the template of the page, we can do the following:
<ion-content>
<ion-list>
<ion-reorder-group (ionItemReorder)="reorderItems($event)" disabled="false">
<ion-item *ngFor="let animal of animals">
<ion-label>
{{animal}}
</ion-label>
<ion-reorder slot="end"></ion-reorder>
</ion-item>
</ion-reorder-group>
</ion-list>
</ion-content>
First inside the ion-content
we will use the ion-list
component, that is made of multiple rows which can contain text, icons and images. The ion-list
will enable us to reorder items within the list.
ionItemReorder
is an event used with component ion-reorder-group
, which will enable us to complete the reordering of the items. The attribute disabled
will show the reorder icon if it is set to false
.
The ion-item
component will contain an ngFor
directive that will loop inside the array animals
and display them in a list.
The ion-label
component will contain the name of each animal in this list.
The attribute slot
of ion-reorder
will specify where the reorder icon should be added, in this example it is added at the end of the item.
After implementing the above, we will get the following:
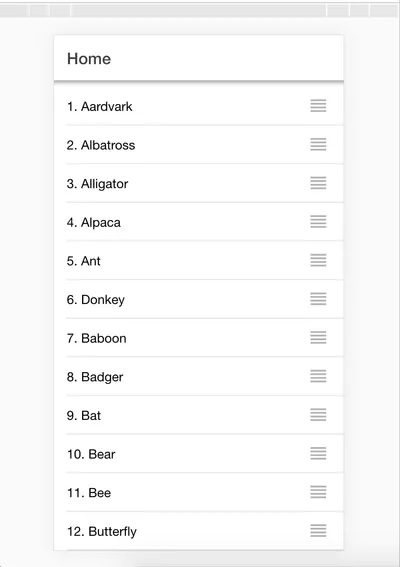
Now, inside the method reorderItems($event)
, we can add the following code:
reorderItems(event)
{
console.log(event);
console.log(`Moving item from ${event.detail.from} to ${event.detail.to}`);
const itemMove = this.animals.splice(event.detail.from, 1)[0];
this.animals.splice(event.detail.to, 0, itemMove);
event.detail.complete();
}
The event's detail
will contain the from
and to
index of the item dragged, adding a console.log
will give the following:
detail:
complete: ƒ ()
from: 0
to: 1
First, we use the splice()
method to remove the dragged item from the array, and assign it to the variable itemMove
. Then, we add the item at the position of event.detail.to
.
After that we can call the method complete()
in order to complete the reorder operation.
Source Code
home.page.ts
:
import { Component } from '@angular/core';
@Component({
selector: 'app-home',
templateUrl: 'home.page.html',
styleUrls: ['home.page.scss'],
})
export class HomePage {
animals : any;
constructor()
{
this.animals =
[
"1. Aardvark",
"2. Albatross",
"3. Alligator",
"4. Alpaca",
"5. Ant",
"6. Donkey",
"7. Baboon",
"8. Badger",
"9. Bat",
"10. Bear",
"11. Bee",
"12. Butterfly",
"13. Camel",
"14. Chicken",
"15. Cockroach",
"16. Horse",
]
}
reorderItems(event)
{
console.log(event);
console.log(`Moving item from ${event.detail.from} to ${event.detail.to}`);
const itemMove = this.animals.splice(event.detail.from, 1)[0];
console.log(itemMove);
this.animals.splice(event.detail.to, 0, itemMove);
event.detail.complete();
}
}
home.page.html
:
<ion-header>
<ion-toolbar>
<ion-title>
Home
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list>
<ion-reorder-group (ionItemReorder)="reorderItems($event)" disabled="false">
<ion-item *ngFor="let animal of animals">
<ion-label>
{{animal}}
</ion-label>
<ion-reorder slot="end"></ion-reorder>
</ion-item>
</ion-reorder-group>
</ion-list>
</ion-content>
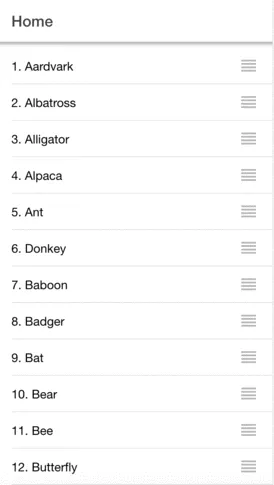
I hope you enjoyed reading this ionic tutorial about reorder, please feel free to leave any comments or feedback on this post!